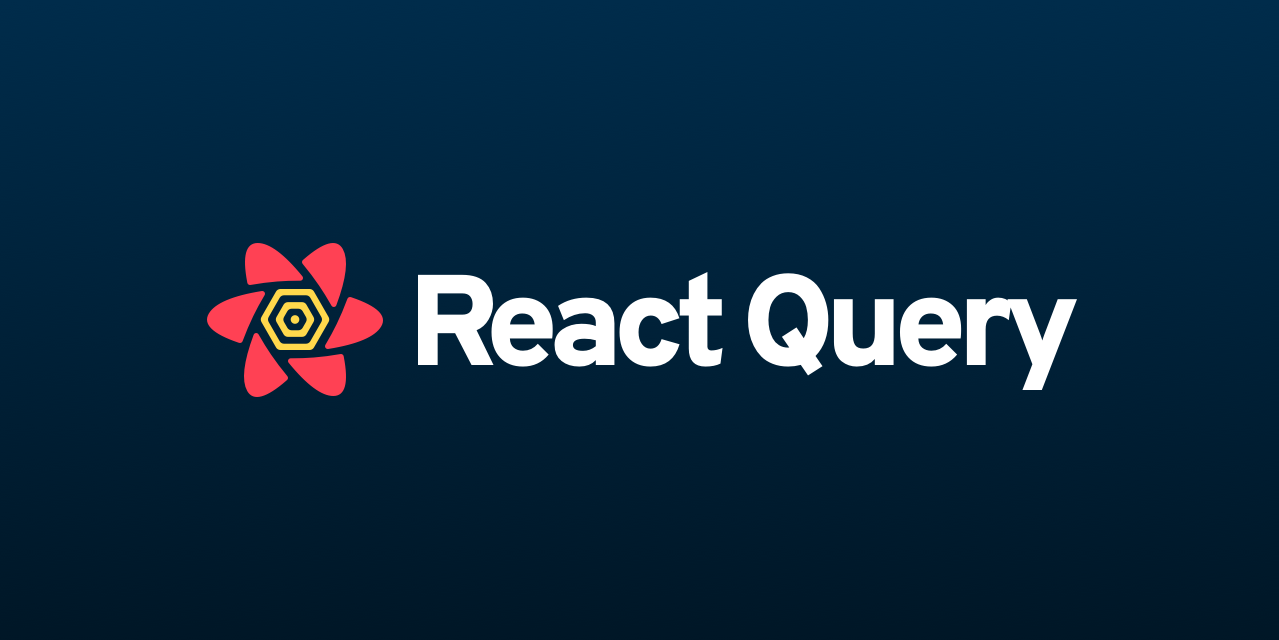
December 6, 2022
Why You Should Start Using React Query Today
React Query is a powerful library that helps developers manage and fetch asynchronous data in React applications. With its intuitive API and seamless integration with React, React Query makes it easy for developers to fetch, cache, and update data in their applications, improving their user experience and performance. In this blog post, we will discuss why developers should consider using React Query in their projects.
React Query overview
A brief overview of React Query. It is a lightweight library for data fetching and caching in React applications. It provides a powerful, yet simple API for fetching, caching, and updating data in an efficient way. React Query is designed to provide an intuitive and declarative way of managing data in React applications. It is optimized for performance, so requests are made in an efficient and fast way, while still providing the flexibility needed to easily update data. React Query also provides a robust API for handling errors, pagination, and more. It is a great solution for managing complex data in React applications.
To start off using React Query, you will need to install the package using either npm or yarn. Then, we can use the React Query hooks to create queries, fetch data, and update the state. You can also use the React Query component to declaratively set up data fetching and caching. Finally, React Query provides a set of utilities that can assist you in managing your data, including caching, pagination, error handling, and more.
How to use React Query to fetch and cache data from an API.
For example, to use React Query to fetch data from an API, you can use the useQuery hook, like this:
import { useQuery } from "react-query"; const fetchUser = async () => { const res = await fetch("https://reqres.in/api/users/2"); return res.json(); }; function App() { const { data, isLoading, error} = useQuery("user", fetchUser); if (isLoading) return "Loading..."; if (error) return "An error has occurred: " + error.message; return ( <div> <h1>{data.name}</h1> <p>{data.description}</p> </div> ); }
This code will create a query that will fetch data from the API and store the result in the query’s context. You can then use the data in your application, as well as the isLoading and error props to handle the loading state and errors.
The benefits of using React Query, such as automatic cache updates and support for pagination and server-side rendering.
When a new instance of useQuery(‘user’, fetchUser) is mounted, a hard loading state is shown and a network request is made to fetch the data. The hook then caches the data and marks itself as stale after the configured staleTime (which is 0 by default).
If a second instance of the same query appears, the data is immediately returned from the cache. A background refetch is triggered for both queries, and if the fetch is successful, both instances are updated with the new data. When the instances are unmounted, a cache timeout is set using cacheTime (defaults to 5 minutes) to delete and garbage collect the query, and if no more instances appear within that time, the query and its data are deleted and garbage collected.
Good set of Developer Tools
React Query Devtools is a powerful set of tools for developers to manage and debug their React Query applications. It provides a comprehensive overview of the current state of the application, as well as detailed information about the data being fetched and the queries being used. It can also be used to debug data fetching, track changes, and identify performance bottlenecks. React Query Devtools is a great tool for any React Query developer because it helps them to quickly identify and fix any issues with their data fetching.

As you can see on the bottom right corner the use of the data explorer helps make sure sure correct queries are being rand and what data is being retrieved.
Advanced features of React Query, such as query invalidation and custom hooks for fetching and updating data.
Query Invalidation
Query invalidation allows React Query to intelligently track the state of data within an application and selectively invalidate queries when the underlying data changes. This allows React Query to avoid unnecessary network requests and ensure that the application is always displaying the most up-to-date data.
Query invalidation is triggered when the underlying data changes. For example, if an application was tracking the stock price of a company, React Query would invalidate any queries related to that data whenever the stock price changed. This would ensure that the application would always be displaying the most up-to-date information about the stock.
Custom Hooks for Fetching and Updating Data
React Query integrates with React Hooks, allowing you to easily access query data in your components. This makes it easier to use in modern React applications. React Query provides powerful custom hooks that enable developers to quickly and easily fetch, update, and manage data within their application. These hooks make it easy to handle common data operations like caching, refetching, pagination, and more. Additionally, React Query provides APIs for customizing the behavior of these hooks, allowing developers to tailor the data fetching and updating experiences to their specific needs.
Common patterns and best practices for using React Query in a real-world application.
If you’re looking toi get started with react query in a real-world application, here are some common patterns and best practices to consider:
Use the in-memory cache option
React Query provides a built-in in-memory cache which can significantly reduce the number of requests you need to make to your backend. This can help reduce the load on your servers and improve the performance of your application.
function App() { const { data, isLoading, error} = useQuery("user", fetchUser, { cacheTime: 0, }); if (isLoading) return "Loading..."; if (error) return "An error has occurred: " + error.message; return ( <div> <h1>{data.name}</h1> <p>{data.description}</p> </div> ); }
Use the query caching feature
React Query offers a query caching feature that allows you to store the results of certain queries in the browser’s localStorage. This can be useful for storing the results of frequent queries so that they don’t need to be fetched from the server every time.
Use the refetchOnWindowFocus option:
The refetchOnWindowFocus option allows you to automatically refetch queries when the user’s focus returns to the page. This can be useful for keeping the data displayed on the page up to date without the user having to manually refresh the page.
function App() { const { data, isLoading, error} = useQuery("user", fetchUser, { refetchOnWindowFocus: false, }); if (isLoading) return "Loading..."; if (error) return "An error has occurred: " + error.message; return ( <div> <h1>{data.name}</h1> <p>{data.description}</p> </div> ); }
Use the polling feature:
React Query’s polling feature can be used to periodically refetch queries in order to keep the data displayed on the page up to date. This can be useful for displaying data that is frequently changing, such as live stock prices or the current weather.
function App() { const { data,isLoading, error } = useQuery("user", fetchUser, { refetchInterval: 1000, }); if (isLoading) return "Loading..."; if (error) return "An error has occurred: " + error.message; return ( <div> <h1>{data.name}</h1> <p>{data.description}</p> </div> ); }
Use the suspense mode:
function App() { const { data,isLoading, error } = useQuery("user", fetchUser, { suspense: true, }); if (isLoading) return "Loading..."; if (error) return "An error has occurred: " + error.message; return ( <div> <h1>{data.name}</h1> <p>{data.description}</p> </div> ); }
React Query’s suspense mode allows you to suspend the rendering of a component until the data that the component needs has been fetched. This can be useful for displaying information that is essential to the page but may take a few seconds to fetch. 6. Use the error handling feature: React Query provides an advanced error handling feature that allows you to easily handle errors that occur when fetching data from your backend. This can be useful for displaying meaningful error messages to the user and for logging errors that occur in production.
Overall Easy to use Library
React Query is a powerful and flexible library for managing asynchronous data in React applications. Its features, such as automatic cache updates and support for pagination and server-side rendering, make it easy to build performant and user-friendly applications. The React Query devtools provide valuable insights into the inner workings of your application, and following best practices for using React Query can help you avoid common pitfalls and take full advantage of its capabilities. Overall, React Query is a valuable tool for any React developer looking to improve the performance and usability of their applications.