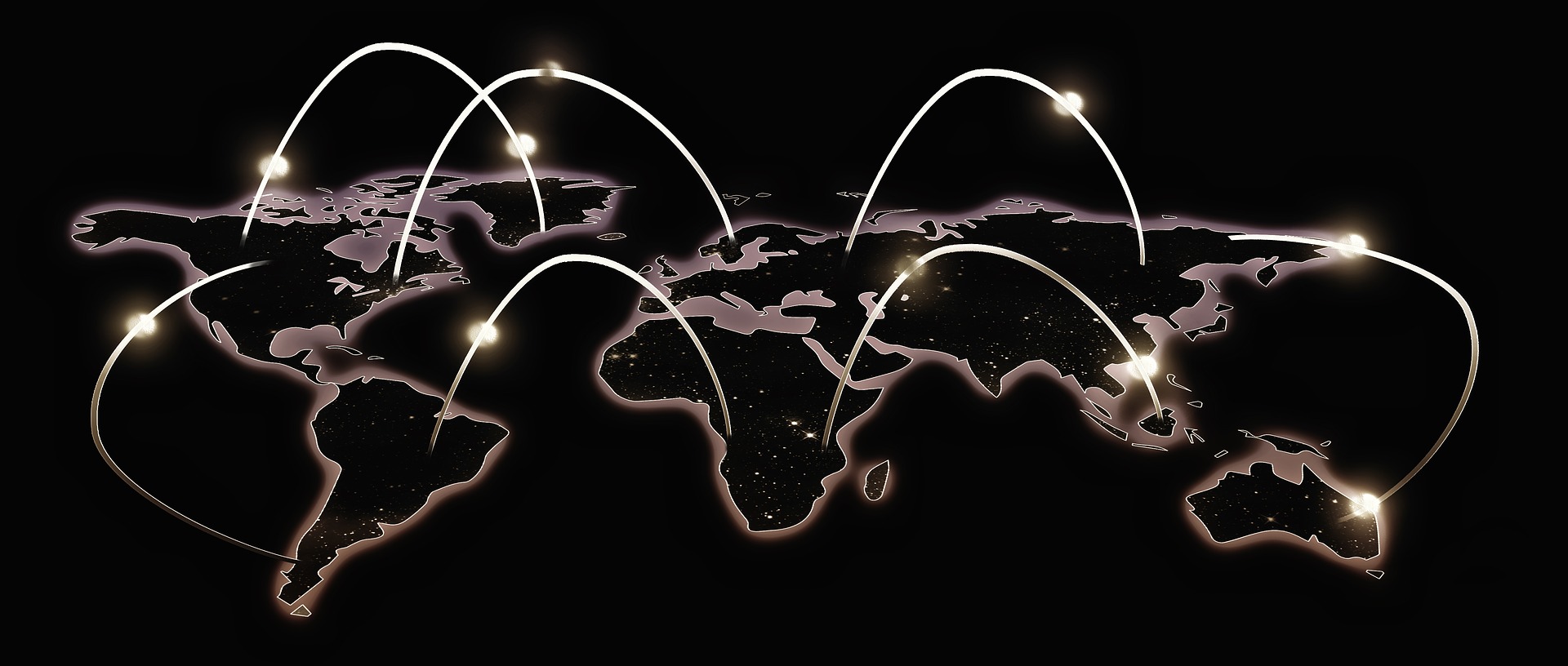
November 28, 2022
The JavaScript Map Object and How to Use it.
The JavaScript Map object is an inbuilt data structure type which helps you to hold an array of values. The main difference between Map and Set is that Map has a key as well as a value, whereas Set only has a value. In JavaScript, the Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value.
The JavaScript map object is an inbuilt data structure type which helps you to hold an array of values.
The JavaScript map object is an inbuilt data structure type which helps you to hold an array of values.
The Map object remembers the original insertion order of the keys, whereas each value can be accessed using its key. This makes maps very useful when you want to look up some specific values based on their key.
JavaScript Maps are mostly used to store primitive values or arrays as keys and corresponding objects as values:
The main difference between Map and Set is that Map has a key as well as a value, whereas Set only has a value.
The main difference between Map and Set is that Map has a key as well as a value, whereas Set only has a value. The key allows you to store more than one value with the same key. Let’s look at an example:
Example in JavaScript:
var map1 = new Map(); map1.set('cat', 'meow'); // 1st item (value = 'meow') map1.set('dog', 'woof'); // 2nd item (value = 'woof')
Map is a collection of keyed data items, just like an Object
. But the main difference is that Map
allows keys of any type.
Methods and properties are:
new Map()
– creates the map.map.set(key, value)
– stores the value by the key.map.get(key)
– returns the value by the key,undefined
ifkey
doesn’t exist in map.map.has(key)
– returnstrue
if thekey
exists,false
otherwise.map.delete(key)
– removes the element (the key/value pair) by the key.map.clear()
– removes everything from the map.map.size
– returns the current element count.
JavaScript Map Objects can also be used as keys
let foo = { n: 1 }; let visitsCountMap = new Map(); visitsCountMap.set(foo, 123); // 123 console.log(visitsCountMap.get(foo));
To reiterate, a Map is a data structure that stores key-value pairs. You can use any type of object as a key, including an object. For now we won’t worry about custom generics.
One of the most notable features of Map is the use of objects as keys. String is also a key in Map, but we cannot use another Map as a key in Map.
var myMap = {}; var key1 = {}; var key2 = {}; myMap[key1] = "value1"; myMap[key2] = "value2"; console.log(myMap); // Output: { '[object Object]': 'value2' }
In JavaScript, the Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value.
The Map object is a data structure that holds key-value pairs. It is one of the most important additions to JavaScript in ES6, or ECMAScript 6. This article will show you how the Map object works and give you some examples on how to use it.
The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value.
Iteration over Map
For looping over a map
, there are 3 methods:
map.keys()
– returns an iterable for keys,map.values()
– returns an iterable for values,map.entries()
– returns an iterable for entries[key, value]
, it’s used by default infor..of
.
const cities = new Map([ ['London', 8615246], ['Berlin', 3562166], ['Madrid', 3165235], ]); //iterate over keys (cities) for (let city of cities.keys()) { console.log(city); } // iterate over values (populations) for (let population of cities.values()) { console.log(population); } // iterate over [key, value] entries for (let entry of cities.entries()) { console.log(entry); } // iterate over [key, value] entries with destructuring for (let [city, population] of cities.entries()) { console.log(city, population); }
Map also has a built in high order array function.
cities.forEach((value, key) => console.log(key + ' = ' + value));
Iterating using for loop
Iterating over keys and values: You can use the keys()
method to get an iterator object that contains all the keys in the Map
object, and the values()
method to get an iterator object that contains all the values. You can then use a loop, such as a for...of
loop, to iterate over the keys or values. For example:
for (const key of map.keys()) { console.log(key); } for (const value of map.values()) { console.log(value); }
Object.fromEntries: Object from Map
The Object.fromEntries() method transforms a list of key-value pairs into an object. This is the inverse of Object.entries(). The syntax is: Object.fromEntries(iterable) Where iterable is an iterable object, such as an Array, Map, Set, etc.
For example, you have a list of key-value pairs:
const arr = [ ['a', 1], ['b', 2], ['c', 3], ];
You can use Object.fromEntries() to create an object from this list: const obj = Object.fromEntries(arr); console.log(obj); // { a: 1, b: 2, c: 3 }
Object.entries: Map from Object
When a Map
is created, we can pass an array (or another iterable) with key/value pairs for initialization.
If we have a plain object, and we’d like to create a Map
from it, then we can use built-in method Object.entries(obj) that returns an array of key/value pairs for an object exactly in that format.
So we can create a map from an object like this:
let obj = { city: "LA", temp: 75 }; let map = new Map(Object.entries(obj)); // LA alert( map.get('city') );
Deleting an Entry
Deleting entries: You can use the delete()
method to remove a specific entry from the Map
object by providing the key. For example:
map.delete('key1'); console.log(map.has('key1')); // Output: false
The delete()
method in JavaScript’s Map
object is used to remove a specific key-value pair from the map. Here are some pros and cons of using the delete()
method:
Pros:
- Easy removal: The
delete()
method provides a simple way to remove a key-value pair from theMap
object. You just need to provide the key as an argument to the method, and it will remove the corresponding entry.
- Efficient memory management: When you delete a key-value pair using the
delete()
method, the memory occupied by that entry is freed up. This can be useful in scenarios where you need to manage memory efficiently.
- No side effects: The
delete()
method only removes the specified key-value pair from theMap
object. It does not affect any other entries or modify the structure of theMap
. This can be advantageous when you want to remove a specific entry without impacting the rest of the map.
Cons:
- No return value: One potential drawback of the
delete()
method is that it does not return the deleted value. If you need to retrieve the value before deleting, you would need to use theget()
method to retrieve the value before callingdelete()
. This can lead to additional code complexity and potential performance overhead.
- Limited use cases: The
delete()
method is specifically designed to remove a single key-value pair from theMap
object. If you need to perform more complex operations, such as deleting multiple entries based on certain conditions, you might need to use other methods or techniques.
Conclusion
We discussed the Map object and how to use it in your code. The JavaScript Map object is an inbuilt data structure type which helps you to hold an array of values. The main difference between Map and Set is that Map has a key as well as a value, whereas Set only has a value. In JavaScript, the Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value