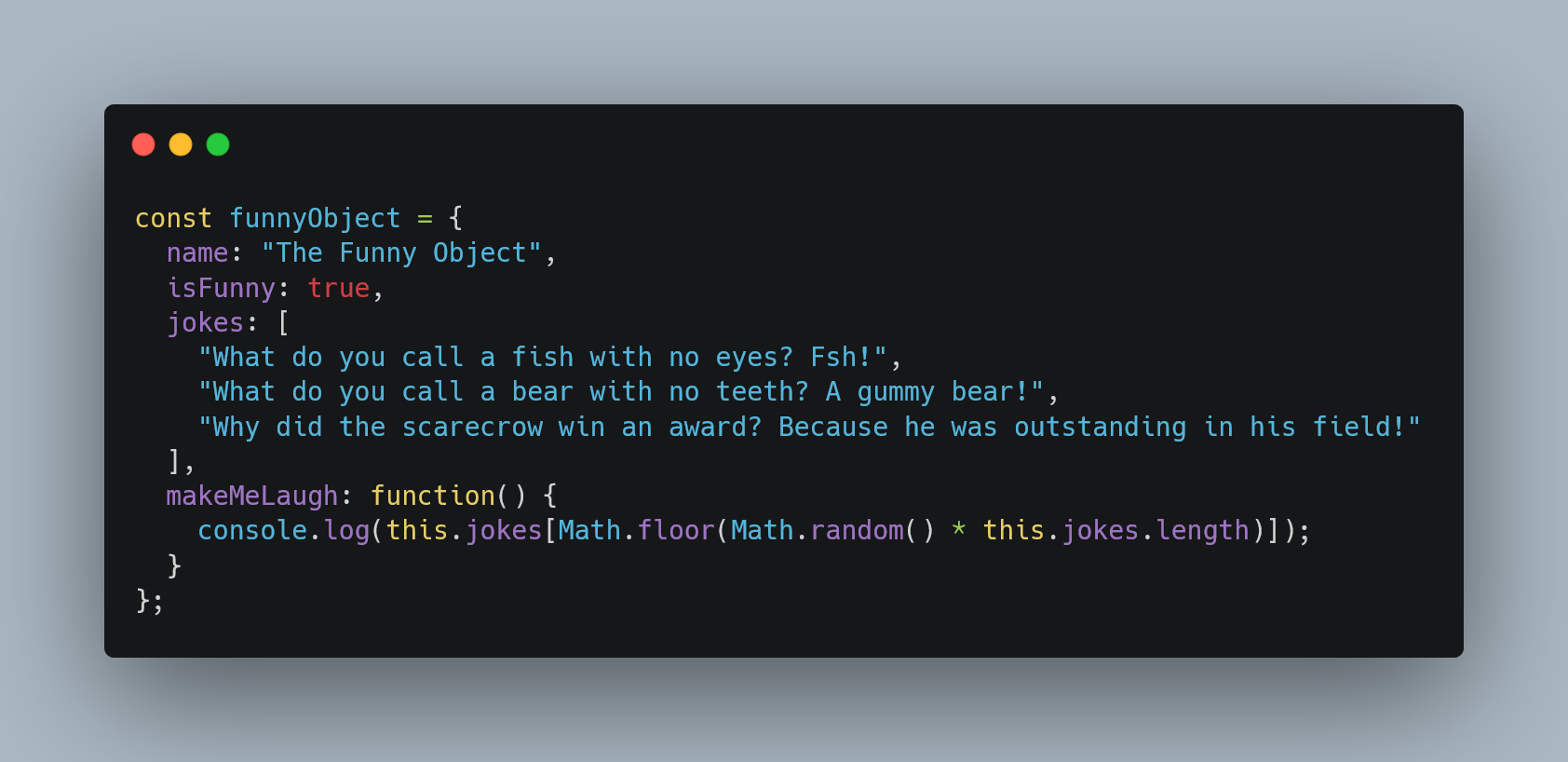
April 25, 2023
In-Depth Guide to Understanding JavaScript Objects
Introduction to JavaScript Objects
JavaScript is a powerful programming language that has been widely used in web development. One of the most important features in JavaScript is its ability to use *objects*. Objects are collections of *properties* that can be accessed and manipulated in a program. JavaScript objects are one of the most powerful features of the language. They allow you to organize your code in a logical way and to reuse code more easily. In this blog post, we will take an in-depth look at JavaScript objects, covering everything from their basic syntax to their advanced features.
What is an Object? In JavaScript, an object
is a data structure that contains key-value pairs. Each key-value pair is referred to as a property of the object. Objects can also contain methods, which are functions that are associated with the object.
Object Literals
One of the simplest ways to create an object in JavaScript is to use an object literal. An object literal is a comma-separated list of key-value pairs wrapped in curly braces.
const person = { name: 'John', age: 30, hobbies: ['reading', 'running', 'swimming'], address: { street: '123 Main St', city: 'New York', country: 'USA' } }; const car = { make: 'Honda', model: 'Civic', year: 2021, isElectric: false, features: ['backup camera', 'sunroof', 'lane departure warning'] };
To manipulate an object in JavaScript, you can access its properties using dot notation or bracket notation. You can also add or remove properties and methods dynamically. .One way to manipulate objects is by accessing its properties using dot notation.
Another way is by using bracket notation. Properties can be added or removed dynamically using object methods like *Object.assign()* or *Object.defineProperty()*. In complex software systems, objects are used to organize and encapsulate code. Understanding how to work with objects is essential for building scalable applications in JavaScript. To become an expert in objects, it’s important to practice creating and manipulating objects using object literals and object methods.
Object Basics
To become proficient in JavaScript objects, it’s essential to understand their basics. Here are some crucial points to keep in mind:
- Objects are collections of key-value pairs that are used to store data.
- Properties in an object can be accessed using dot notation or bracket notation.
- Objects can contain methods, which are functions that perform actions on the object.
- Object literals are a simple and easy way to create objects in JavaScript.
- In complex software systems, objects are used to organize and encapsulate code.
To further master the use of objects, it’s important to practice creating and manipulating objects. This will help you build scalable applications in JavaScript.
How to access object properties and methods
Here’s an example of accessing properties using dot notation:
const person = { name: 'John', age: 30 }; console.log(person.name); // Output: John
And here’s an example of accessing properties using bracket notation:
const person = { name: 'John', age: 30 }; console.log(person['name']); // Output: John
To access methods of an object, you can use dot notation as well:
const person = { name: 'John', age: 30, greet() { console.log(`Hello, my name is ${this.name}`); } }; person.greet(); // Output: Hello, my name is John
How to use objects with functions
To use objects with functions, you can pass an object as a parameter to a function. Here is an example:
function printPerson(person) { console.log(`Name: ${<a target="_blank" rel="noreferrer noopener" href="http://person.name">person.name</a>}, Age: ${person.age}`); } const john = { name: 'John', age: 30 }; printPerson(john); // Output: Name: John, Age: 30
You can also return an object from a function. Here is an example:
function createPerson(name, age) { return { name, age }; } const john = createPerson('John', 30); console.log(john); // Output: { name: 'John', age: 30 }
Using objects with functions is a useful approach in JavaScript programming. It allows you to organize and manipulate data in a more intuitive way. When passing objects as parameters or returning them from functions, you can use dot notation or bracket notation to access their properties and methods. By practicing with object literals and object methods, you can become proficient in working with objects and build scalable applications with ease. Remember that objects are crucial tools in JavaScript programming and understanding them is essential to becoming a proficient developer.
How to use objects with the DOM
To use objects with the DOM, you can create an object to represent the elements on a web page, and then manipulate them using object methods. Here’s an example:
const myButton = { element: document.querySelector('#my-button'), text: 'Click me', onClick() { console.log('Button clicked!'); }, updateText(newText) { this.text = newText; this.element.innerText = newText; } }; myButton.element.addEventListener('click', () => { myButton.onClick(); }); myButton.updateText('New button text');
In this example, `myButton` is an object that represents a button element on a web page. It has properties for the button’s element,text, and methods for handling button clicks and updating the button’s text. The object methods are called using dot notation and are associated with the `myButton` object. By representing web page elements as objects, you can write more modular and reusable code. This is a fundamental aspect of JavaScript programming, where you can use objects to create powerful and object-oriented applications. By practicing with objects and the DOM, you can build complex and interactive web applications.
How to use objects with promises
Objects can be used with promises in JavaScript to store and manipulate data that is asynchronously retrieved or processed. Promises are objects that represent the eventual completion or failure of an asynchronous operation and provide a way to handle the result or error when it becomes available. By storing data in objects, you can easily pass it between functions or modules and update it as needed when promises resolve or reject. You can also use objects to define and configure Promises, such as setting the timeout or specifying a fallback value in case of errors. Objects and promises can work together to create powerful and flexible code that can handle complex asynchronous operations.
const myObj = { fetchData: function() { return new Promise((resolve, reject) => { // code to fetch data from an API or database const data = {foo: 'bar'}; if (data) { resolve(data); } else { reject(Error('Data not found')); } }); } }; myObj.fetchData() .then(data => { console.log(data); // {foo: 'bar'} }) .catch(error => { console.error(error); // Error: Data not found });
In this example, we have an object called myObj
with a method called fetchData
that returns a Promise. The Promise resolves with some data (in this case, an object with a foo
property set to the string 'bar'
) if the data is successfully fetched, or rejects with an Error if the data is not found.
We can then use this object and its Promise-returning method to fetch and use the data in our application. We call the fetchData
method on myObj
, and then use .then()
to handle the resolved Promise (where we log the data to the console), and .catch()
to handle any rejected Promises (where we log the Error to the console).
JavaScript objects are a fundamental aspect of the language that allow developers to organize and manipulate data in a more intuitive and efficient way. Whether you are working with the DOM, functions, or promises, objects can help you write more modular and reusable code. By becoming proficient in working with objects, you can build powerful and object-oriented applications that are scalable and easy to maintain. Remember to practice with object literals and object methods, and to keep in mind the basics of working with objects, such as accessing properties and methods using dot notation or bracket notation. With these skills, you can take your JavaScript programming to the next level and create complex and interactive web applications.